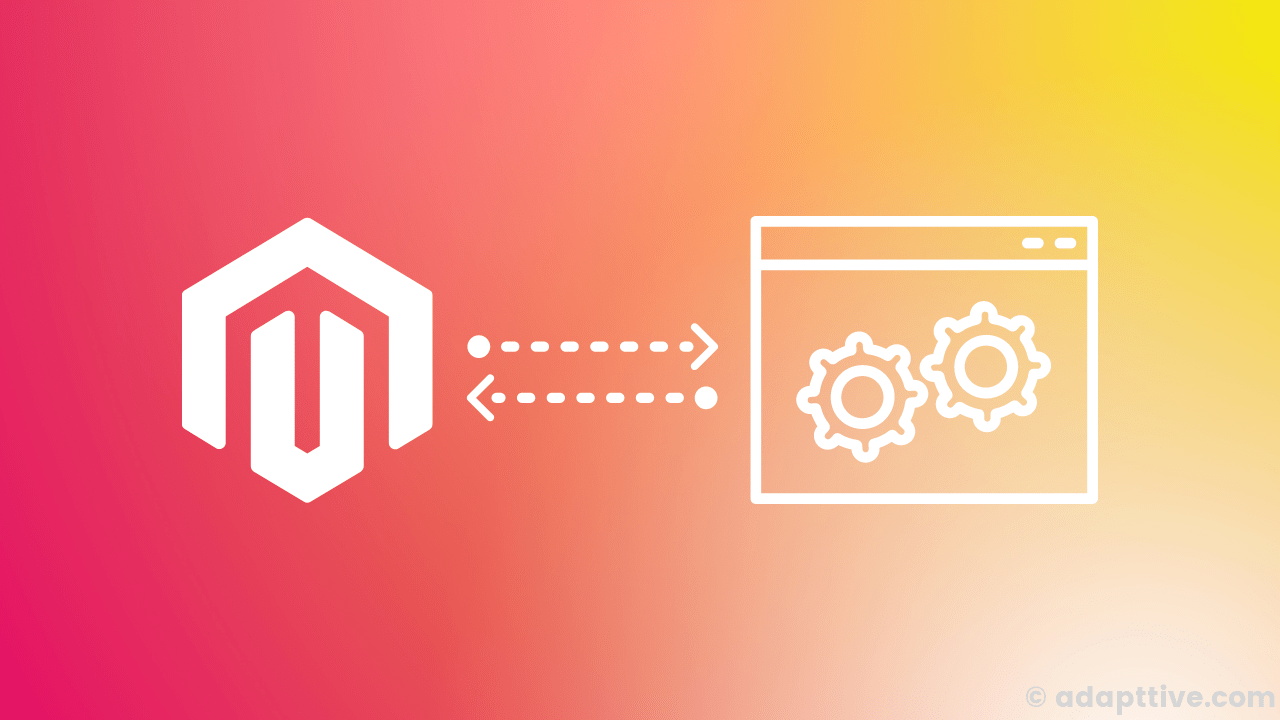
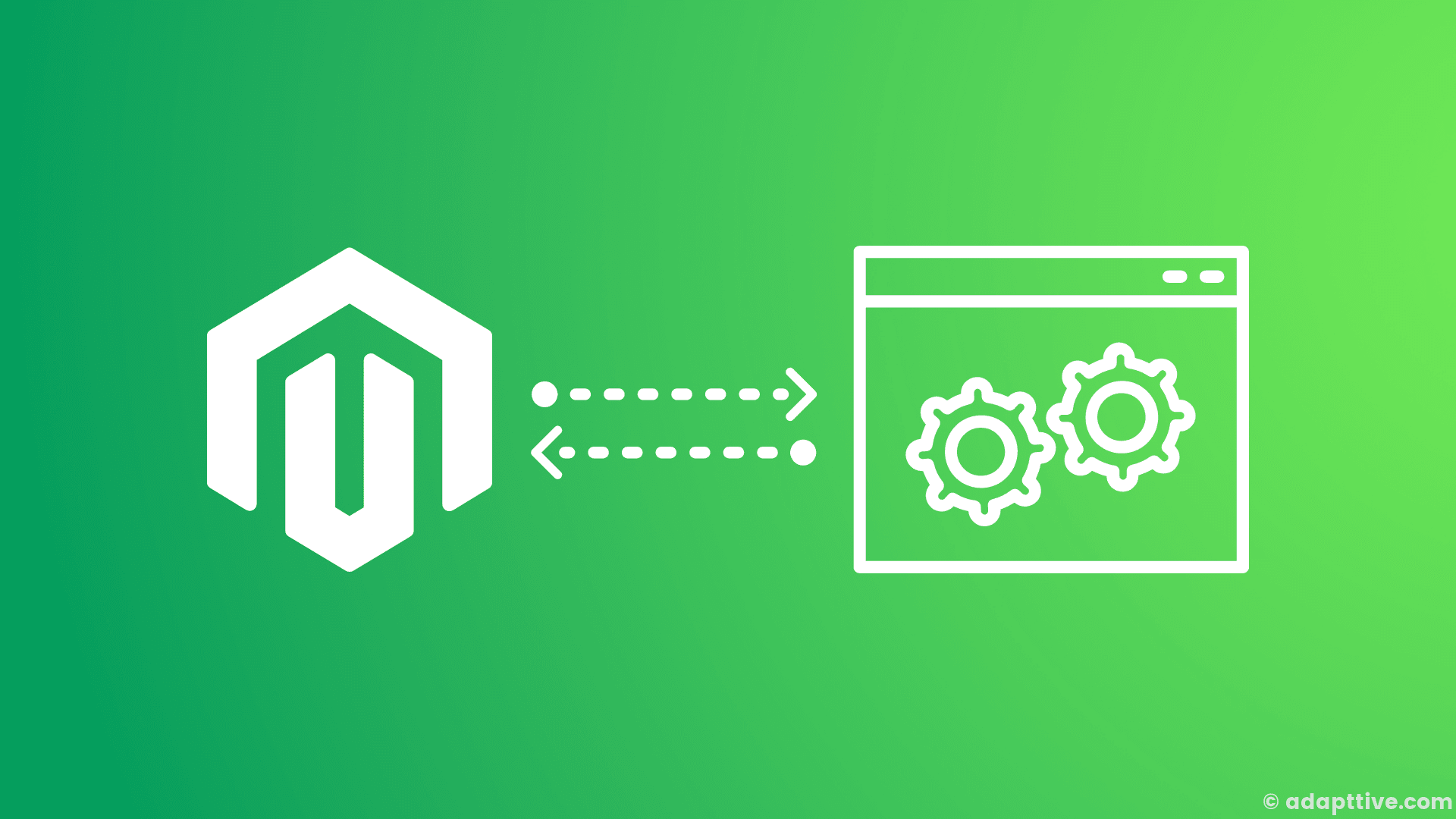
Find order id (entity_id) from increment id in Magento 2 using a lightweight DB query without loading the complete order model.
In this article, I will show you the best (optimized) way to get order id ie sales_order.entity_id
from increment id by creating a custom DB query in Magento2.
-
Why do you need order id?
-
Order state change requires order id. Example:
\Magento\Sales\Api\OrderManagementInterface::cancel($id)
\Magento\Sales\Api\OrderManagementInterface::hold($id)
\Magento\Sales\Api\OrderManagementInterface::unHold($id)
-
Get order records. Example:
\Magento\Sales\Api\OrderRepositoryInterface::get($id)
-
-
How can we do this?
- Create
GetOrderIdByIncrementId
:
<?php declare(strict_types =1); namespace Adapttive\SalesOrder\Model\Order; use Exception; use Magento\Sales\Model\ResourceModel\Order as OrderResource; /** * Class GetOrderIdByIncrementId: To get order.entity_id by order.increment_id * Reference from \Magento\Sales\Model\OrderIncrementIdChecker */ class GetOrderIdByIncrementId { /** * @var OrderResource */ private $resource; /** * @param OrderResource $resource */ public function __construct(OrderResource $resource) { $this->resource = $resource; } /** * Get order id by order increment id. * * @param string|int $incrementId * @return int * @throws \Magento\Framework\Exception\LocalizedException */ public function get($incrementId): int { $result = 0; try { /** @var \Magento\Framework\DB\Adapter\AdapterInterface $adapter */ $adapter = $this->resource->getConnection(); $bind = [':increment_id' => $incrementId]; /** @var \Magento\Framework\DB\Select $select */ $select = $adapter->select(); $select->from($this->resource->getMainTable(), $this->resource->getIdFieldName()) ->where('increment_id = :increment_id'); $entityId = $adapter->fetchOne($select, $bind); if ($entityId > 0) { $result = (int)$entityId; } } catch (Exception $e) { $result = 0; } return $result; } }
- Usage:
$orderId = $this->getOrderIdByIncrementId->get($orderIncrementId)
- Create